PHP Data Types and Operators
PHP Data Types
Variables can store data of different types, and different data types can do different things.
PHP supports the following data types:
- String
- Integer
- Float (floating point numbers - also called double)
- Boolean
- Array
- Object
- NULL
- Resource
PHP String
A string is a sequence or arrangement of characters known as string, like "Hello testing!".
<?php
$x = 'Hello world!';
$y = 'Hello world!'; //we can also use double quote
echo $x;
echo '';
echo $y;
?>
Output : Hello world! Hello world!
PHP Integer
An integer data type is a non-decimal number between -2,147,483,648 and 2,147,483,647.
<?php
$x = 10;
var_dump($x);
?>
output: int(10)
PHP Float
A float (floating point number) is a number with a decimal point or a number in exponential form.
<?php
$x = 10.365;
var_dump($x);
?>
Output: float(10.365)
PHP Boolean
This type only True & False
PHP Array
<?php
$al= array("A","B","C");
var_dump($al);
?>
PHP Object
Object is an instantiation of a class
<?php
//Car is a class
class Car {
public $color;
public $model;
public function __construct($color, $model) {
$this->color = $color;
$this->model = $model;
}
public function message() {
return "My car is a " . $this->color . " " . $this->model . "!";
}
}
// $myCar is an object
$myCar = new Car("black", "Volvo");
echo $myCar -> message();
echo "
";
$myCar = new Car("red", "Toyota");
echo $myCar -> message();
?>
PHP NULL Value
Null is a special data type which can have only one value: NULL.
<?php
$x = "Hello test!";
$x = null;
var_dump($x);
?>
PHP Resource
The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP.
PHP Operators
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
1- Arithmetic operators
Operator | Name | Example | Result |
+ | Addition | $x + $y | Sum of $x and $y |
- | Subtraction | $x - $y | Difference of $x and $y |
* | Multiplication | $x * $y | Product of $x and $y |
/ | Division | $x / $y | Quotient of $x and $y |
% | Modulus | $x % $y | Remainder of $x divided by $y |
** | Exponentiation | $x ** $y | Result of raising $x to the $y'th power |
2- PHP Assignment Operators
Assignment | Same | Description |
x = y | x = y | The left operand gets set to the value of the expression on the right |
x += y | x = x + y | Addition |
x -= y | x = x - y | Subtraction |
x *= y | x = x * y | Multiplication |
x /= y | x = x / y | Division |
x %= y | x = x % y | Modulus |
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
Operator | Name | Example | Result |
== | Equal | $x == $y | Returns true if $x is equal to $y |
=== | Identical | $x === $y | Returns true if $x is equal to $y, and they are of the same type |
!= | Not equal | $x != $y | Returns true if $x is not equal to $y |
<> | Not equal | $x <> $y | Returns true if $x is not equal to $y |
!== | Not identical | $x !== $y | Returns true if $x is not equal to $y, or they are not of the same type |
> | Greater than | $x > $y | Returns true if $x is greater than $y |
< | Less than | $x < $y | Returns true if $x is less than $y |
>= | Greater than or equal to | $x >= $y | Returns true if $x is greater than or equal to $y |
<= | Less than or equal to | $x <= $y | Returns true if $x is less than or equal to $y |
<=> | Spaceship | $x <=> $y | Returns an integer less than, equal to, or greater than zero, depending on if $x is less than, equal to, or greater than $y. Introduced in PHP 7. |
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
Operator | Name | Description |
++$x | Pre-increment | Increments $x by one, then returns $x |
$x++ | Post-increment | Returns $x, then increments $x by one |
--$x | Pre-decrement | Decrements $x by one, then returns $x |
$x-- | Post-decrement | Returns $x, then decrements $x by one |
PHP Logical Operators
Operator | Name | Example |
and | And | $x and $y |
or | Or | $x or $y |
xor | Xor | $x xor $y |
&& | And | $x && $y |
|| | Or | $x || $y |
! | Not | !$x |
PHP Conditional Assignment Operators
Operator | Name | Example |
?: | Ternary | $x = expr1 ? expr2 : expr3 |
?? | Null coalescing | $x = expr1 ?? expr2 |
Related post
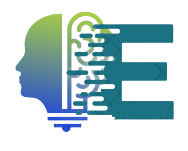
EknowledgePoint
Increase Your Knowledge with usEknowledgePoint is an innovative online learning platform that aims to democratize education by providing a vast array of courses and educational content to learners of all ages and backgrounds. It offers a diverse range of subjects, from academic topics to practical skills, making it a one-stop destination for anyone looking to expand their knowledge base.
5 comments
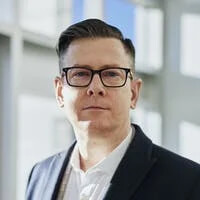
Knowledge is the fuel that powers our journey through life. It's platforms like EknowledgePoint that transform information into enlightenment, curiosity into competence, and dreams into reality. Embrace the opportunity to learn, and you'll find that the doors of possibility swing wide open. 🚀💡 #EknowledgePoint.
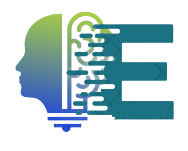
Thank you for your kind words! At EknowledgePoint, we are dedicated to empowering individuals on their learning journey. Your enthusiasm for knowledge and personal growth inspires us to keep providing the best educational resources and experiences. Let's continue to explore and learn together!.
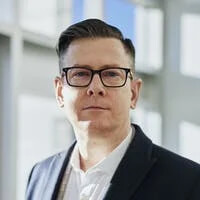
Thanks For Your Support.
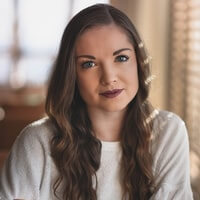
"I'm constantly amazed by the quality of instructors on EknowledgePoint. Learning from experts in their fields is both enlightening and empowering. 🌟👨🏫 #EknowledgePoint.
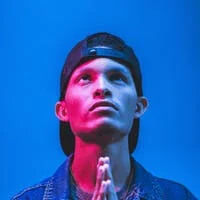
EknowledgePoint isn't just a platform; it's a community of learners. The support and camaraderie here are truly inspiring. 🤝🌍 #EknowledgePoint.